Getting basic animations into Godot Engine is simple and a joy. It is stupidly straight forward.
So first off create a new scene and add an AnimatedSprite node. If you can’t find it, just do a search:
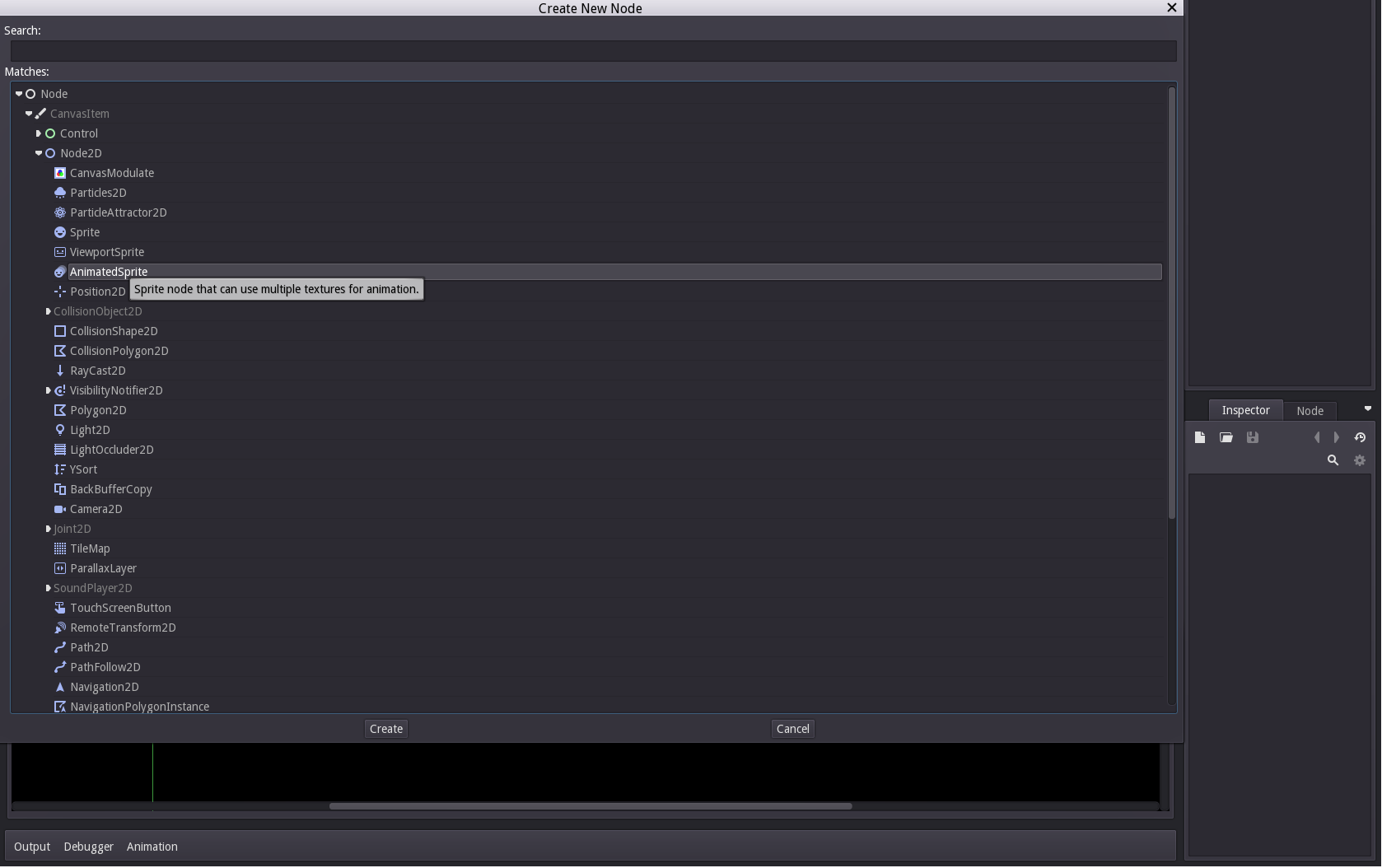
On the right hand-side where the inspector is you’ll see “Frames
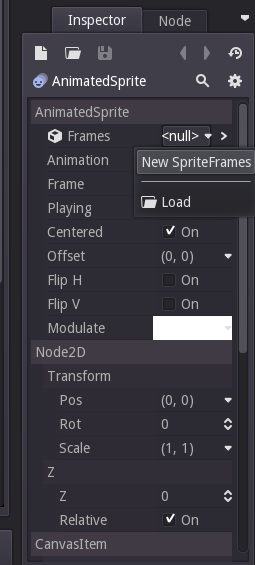
This should open up an animation panel at the bottom. First though you need import your Sprites/images. Importing them as Textures is not required but unless you do, the images will be filtered. If you’re doing pixel art, then you’ll want to import them like so:
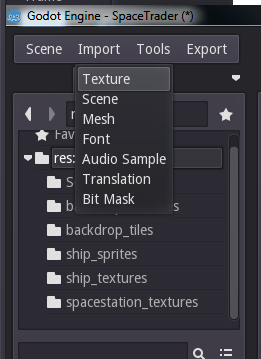
You’ll then be greeted with this popup:
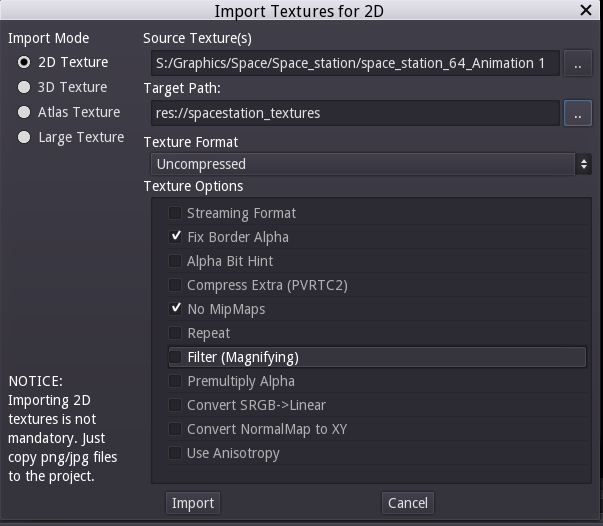
Make sure you select 2D Texture and make sure to disable “Filter (Magnifying)”. Leave the rest as is. If you want, create a new folder to write the Textures to, but having them in the root of your res:// is fine too.
Once this is done, simply select and drag the textures from the FileSystem browser into the Animation Frame at the bottom of the screen:
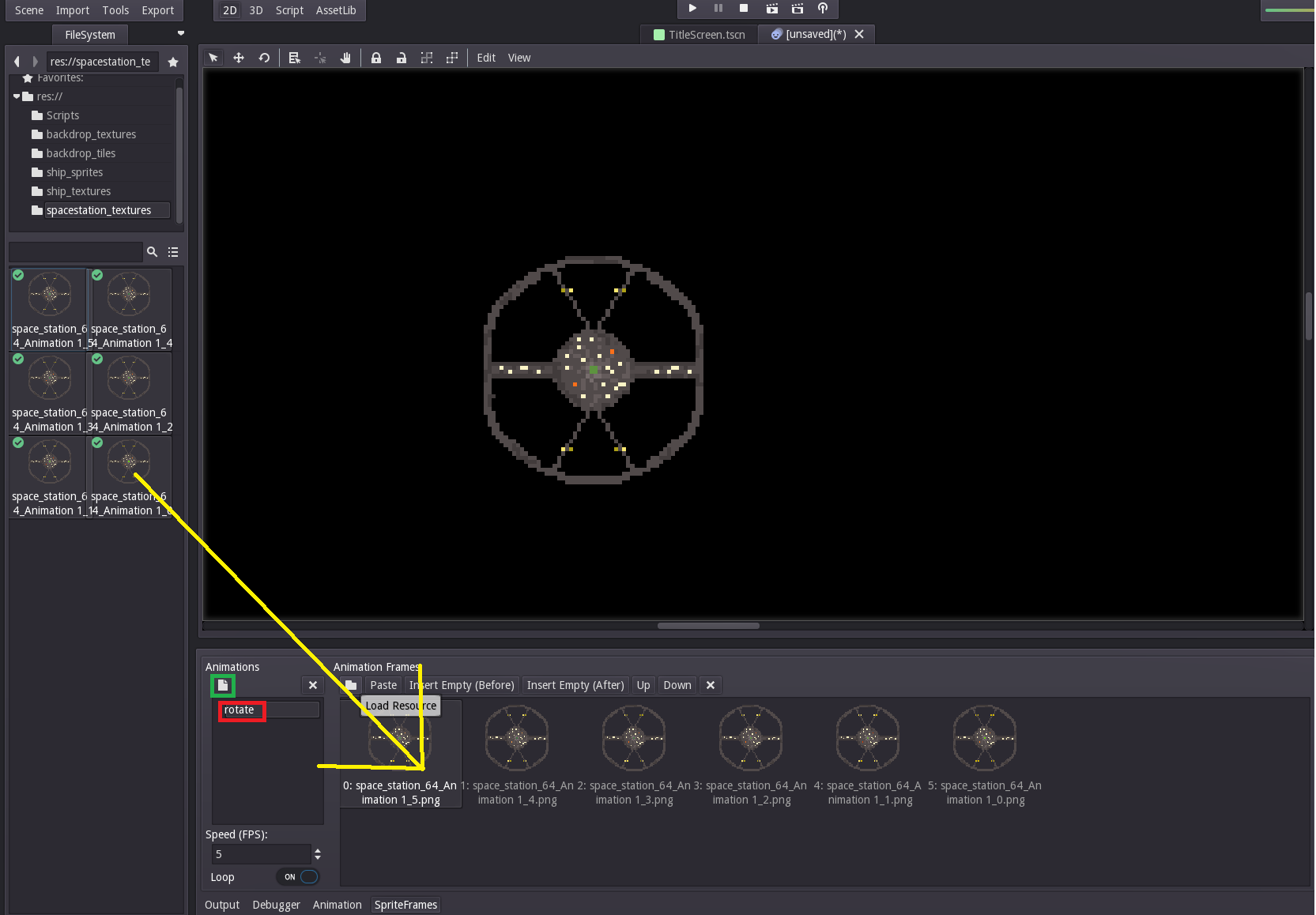
The image above shows that they will be part of an animation called “rotate”(red). You can create more animations by simply clicking the paper icon below animations (green). The name given in the red box is how you can refer to different animations in your scripts.
So now that the new animated node/scene has been created its just a matter of putting it into the main game scene. To do this, simply navigate back to the scene in which you want to put your new animated sprite/node/scene:
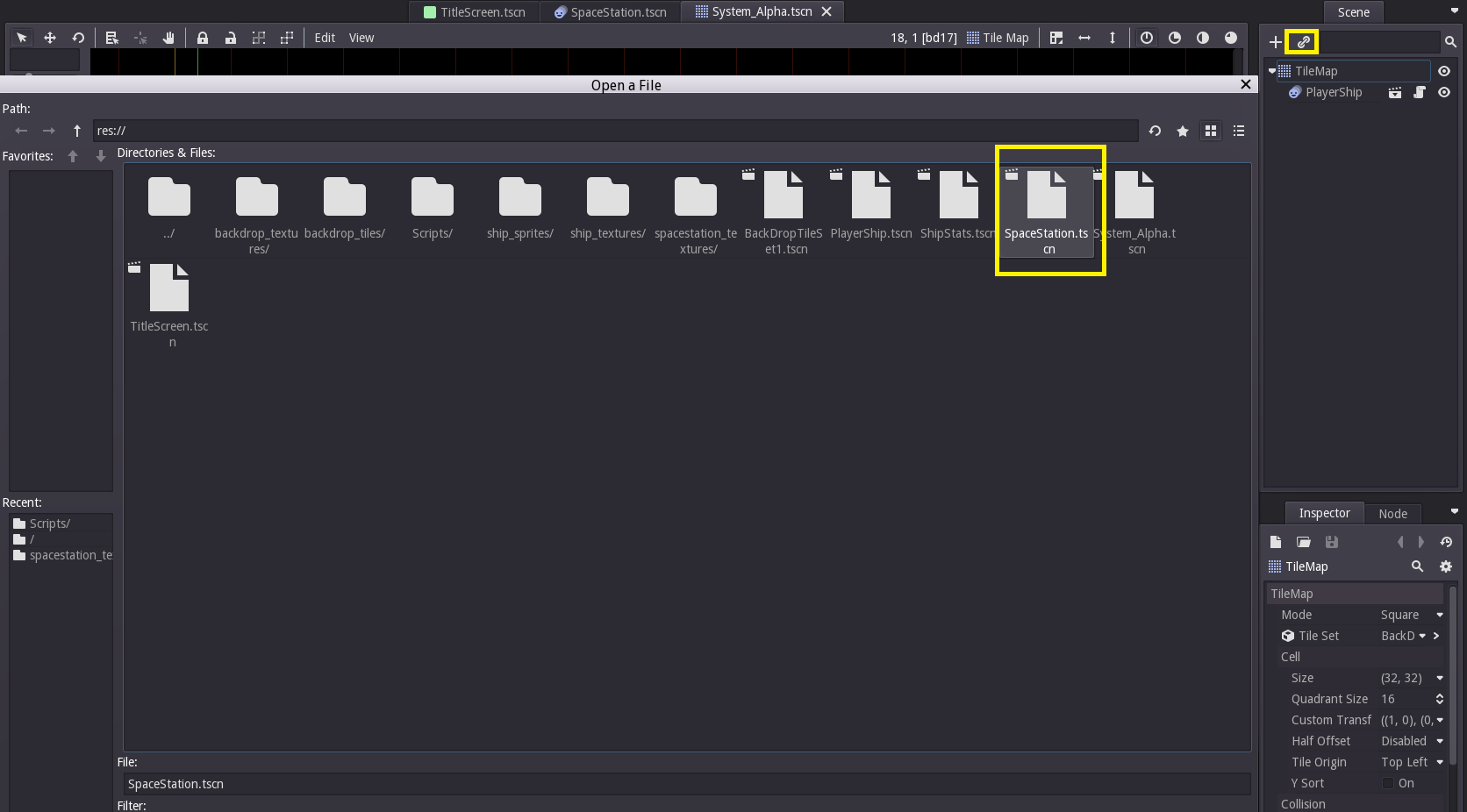
Clicking on the exisiting node you want to parent the instance node to, then click the icon highlighted in yellow in the scene tab. Select the scene and press okay.
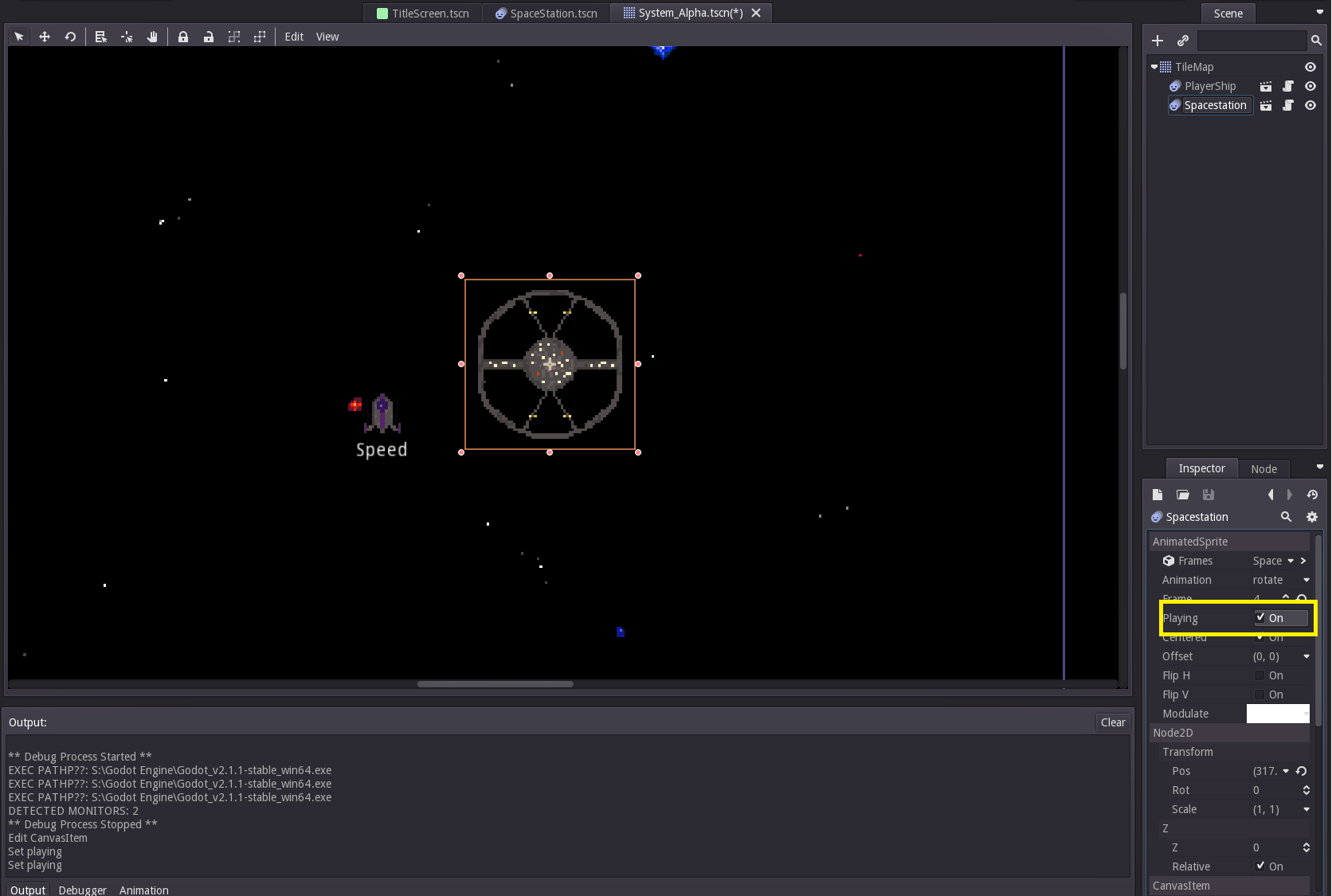
The newly animated scene has now been instanced in the level with the player object. The yellow box shows where you can toggle the playing of the animation in the scene view.